《Revit二次开发官方教程》里的代码适合练手以及功能测试,但是在实际开发中,这种代码肯定不符合开发公司的标准。
这里可以使用这个简单的标准模板,这个标准模板的主要参考revit的SDK的Samples案例以及我自己在公司开发的实践来写的。逻辑很简单,主程序类实例化窗口,一个类用来存储临时数据,然后就是主窗口。
首先来看看winform:
1、主程序类
using System;
using System.Collections.Generic;
using System.Text;
using System.Windows.Forms;
using Autodesk.Revit;
using Autodesk.Revit.DB;
using Autodesk.Revit.UI;
using Autodesk.Revit.DB.Structure;
namespace RevitTest
{
/// <summary>
/// Implements the Revit add-in interface IExternalCommand
/// </summary>
[Autodesk.Revit.Attributes.Transaction(Autodesk.Revit.Attributes.TransactionMode.Manual)]
[Autodesk.Revit.Attributes.Journaling(Autodesk.Revit.Attributes.JournalingMode.NoCommandData)]
public class Command : IExternalCommand
{
public Result Execute(ExternalCommandData commandData, ref string message, ElementSet elements)
{
try
{
//prepare data
TempData tempData = new TempData(commandData);
// show UI
using (ElementIdForm displayForm = new ElementIdForm(tempData))
{
DialogResult result = displayForm.ShowDialog();
if (DialogResult.OK != result)
{
return Autodesk.Revit.UI.Result.Cancelled;
}
}
return Autodesk.Revit.UI.Result.Succeeded;
}
catch (Exception e)
{
message = e.Message;
return Autodesk.Revit.UI.Result.Failed;
}
}
}
}
2、临时数据
using System;
using System.Collections;
using System.Collections.Generic;
using System.Text;
using Autodesk.Revit.DB;
using Autodesk.Revit.UI;
using Autodesk.Revit;
using Autodesk.Revit.UI.Selection;
namespace RevitTest
{
public class TempData
{
private UIDocument m_revitDoc;
private Document m_Doc;
private ElementId m_elemId;
public ElementId ElemId
{
get
{
return m_elemId;
}
set
{
m_elemId = value;
}
}
public TempData(ExternalCommandData commandData)
{
m_revitDoc = commandData.Application.ActiveUIDocument;
m_Doc = commandData.Application.ActiveUIDocument.Document;
Reference reference = m_revitDoc.Selection.PickObject(ObjectType.Element);
m_elemId = m_Doc.GetElement(reference).Id;
}
}
}
3、最后是主窗口
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace RevitTest
{
public partial class ElementIdForm : Form
{
private TempData m_tempData;
public ElementIdForm(TempData tempData)
{
InitializeComponent();
m_tempData= tempData;
textBox1.Text = m_tempData.ElemId.ToString();
}
}
}
然后,换成wpf窗口的简单模板:
主程序代码:
using System;
using System.Collections.Generic;
using System.Text;
using Autodesk.Revit;
using Autodesk.Revit.DB;
using Autodesk.Revit.UI;
using Autodesk.Revit.DB.Structure;
namespace WpfTest
{
/// <summary>
/// Implements the Revit add-in interface IExternalCommand
/// </summary>
[Autodesk.Revit.Attributes.Transaction(Autodesk.Revit.Attributes.TransactionMode.Manual)]
[Autodesk.Revit.Attributes.Journaling(Autodesk.Revit.Attributes.JournalingMode.NoCommandData)]
public class Command : IExternalCommand
{
public Result Execute(ExternalCommandData commandData, ref string message, ElementSet elements)
{
try
{
//prepare data
TempData tempData = new TempData(commandData);
// show UI
ElementIdWindow EIwindow = new ElementIdWindow(tempData);
if(EIwindow.ShowDialog()==true)
{
//DoSomething
}
}
catch (Exception e)
{
message = e.Message;
return Autodesk.Revit.UI.Result.Failed;
}
return Autodesk.Revit.UI.Result.Succeeded;
}
}
}
临时数据
using System;
using System.Collections;
using System.Collections.Generic;
using System.Text;
using Autodesk.Revit.DB;
using Autodesk.Revit.UI;
using Autodesk.Revit;
using Autodesk.Revit.UI.Selection;
namespace WpfTest
{
public class TempData
{
private UIDocument m_revitDoc;
private Document m_Doc;
private string m_elemId;
public string ElemId
{
get
{
return m_elemId;
}
set
{
m_elemId = value;
}
}
public TempData(ExternalCommandData commandData)
{
m_revitDoc = commandData.Application.ActiveUIDocument;
m_Doc = commandData.Application.ActiveUIDocument.Document;
Reference reference = m_revitDoc.Selection.PickObject(ObjectType.Element);
m_elemId = m_Doc.GetElement(reference).Id.ToString();
}
}
}
主窗口代码:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Imaging;
using System.Windows.Navigation;
using System.Windows.Shapes;
namespace WpfTest
{
/// <summary>
/// ElementIdWindow.xaml 的交互逻辑
/// </summary>
public partial class ElementIdWindow : Window
{
public ElementIdWindow(TempData tempData)
{
InitializeComponent();
DataContext = tempData;
}
private void btnOK_Click(object sender, RoutedEventArgs e)
{
this.DialogResult = true;
}
}
}
xaml代码:
<Window x:Class="WpfTest.ElementIdWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:local="clr-namespace:WpfTest"
mc:Ignorable="d" Height="150" Width="200"
d:DesignHeight="300" d:DesignWidth="300">
<Grid>
<StackPanel>
<TextBox Margin="10" Text="{Binding Path=ElemId}"></TextBox>
<Button Margin="10" Click="btnOK_Click">OK</Button>
</StackPanel>
</Grid>
</Window>
其实wpf也挺好用的,特别是绑定数据这个强大的功能。
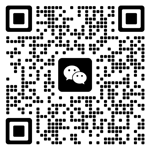
微信公众号:xuebim
关注建筑行业BIM发展、研究建筑新技术,汇集建筑前沿信息!
← 微信扫一扫,关注我们+
评论前必须登录!
注册